WordPress thumbnails (or Featured Images) are a great way to show a single image from a lot of posts at once. This has a lot of applications but the most popular is probably the related posts plugin. You know what I’m talking about, it’s usually at the bottom of a post on any given blog and has 3+ other posts that you might be interested in. Usually just with an image and the post title.
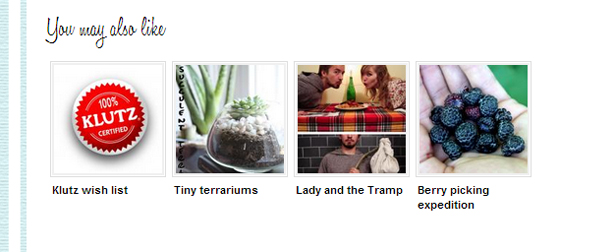
Featured posts plugins use the featured image from any given post rather than crawling each post to try to find the first image in the individual post. Oftentimes this won’t work out of the box if you’re using or creating a custom theme because the post thumbnail functionality needs to be added in your functions.php.
Step 1. Adding the theme functionality
It’s simple, really. First look in your functions.php for this line:
add_theme_support( 'post-thumbnails' );
If it’s there, awesome, move to the next step. If you can’t find it, simply add the following:
if ( function_exists( 'add_theme_support' ) ) { add_theme_support( 'post-thumbnails' ); set_post_thumbnail_size( 150, 150, true ); // Set your thumbnail size here, this will resize then crop. }
This little chunk of code checks to see that the add_theme_support function exists and if it does, creates theme support for the post thumbnails. Pretty self explanatory.
Step 2. Adding thumbnails to your site
So you have thumbnail functionality, now what? Well if you just wanted to use them for related posts then download a plugin like nRelate and be on your way! If you’re looking for something more detailed, such as displaying the post thumbnail next to each post in the blogroll, then you’ll need to add something to the loop.
Adding the Featured Image next to each post
To add the Featured Image/thumbnail next to each post in the loop, first you have to decide which page you want it on. Do you want to have the images display on the ‘standard’ blogroll? If so, you’ll be editing ‘index.php.’ Do you want to have the images display only on the category pages? If so, you’ll just edit ‘category.php.’
Once you know what page you want to edit, open it up. You’ll want to place your thumbnail code somewhere between:
<?php while (have_posts()) : the_post(); ?>
and:
<?php endwhile; ?>
Once you’ve found the proper spot to add it (I tend to add it immediately after the opening while()
statement), just add the following:
<?php echo get_the_post_thumbnail($page->ID, array(150,150)); ?>
If you want to change the size of the image, you’re free to do so here, just edit the values in the array (width,height).
So now your index.php or category.php loop should look something like the following:
<?php if (have_posts()) : ?> <?php while(have_posts()) : the_post(); ?> <div class="post"> <?php echo get_the_post_thumbnail($page->ID, array(150,150)); ?> <h2><?php the_title(); ?></h2> <?php the_excerpt(); ?> </div> <?php endwhile; ?> <?php endif; ?>
Clearly this is a very stripped down loop, but you get the gist.
Adding a Featured Image to each post page
Sometimes if you order articles through a third party such as Brafton, they’ll automatically assign a featured image without putting any images in the blog content itself. This could cause confusion in your readers when they see images with the posts either through your related posts plugin, or next to the articles as I showed above, but then they visit the post itself and there is nothing but text.
So what’s a poor sucker to do? Go through each post individually and add links to all of the images one by one? No way, thankfully you can output the featured image directly on the post page. It’s actually remarkably similar to how we did it earlier. The only difference is that you’ll be editing ‘single.php’ and you’ll probably want to increase the image size so it makes more sense as the single image on the page. So your ‘single.php’ should end up looking something like this:
<?php if (have_posts()) : ?> <?php while(have_posts()) : the_post(); ?> <div> <?php echo get_the_post_thumbnail($page->ID, 'medium'); ?> <h1><?php the_title(); ?></h1> <?php the_content(); ?> </div> <?php endwhile; ?> <?php endif; ?>
The primary difference here is that I’ve used a keyword (medium) rather than putting in the dimensions. Either way works though. You can read more about the featured image/post thumbnails in the Codex. As always, drop any questions below!